In this article I am going to calculate distance using HC-SR04 and raspberry pi.
The HC-SR04 Ultrasonic sensor that I am going to use here has four pins:
- Ground (GND)
- Echo Pulse Output (ECHO)
- Trigger Pulse Input (TRIG)
- and 5V Supply (Vcc)
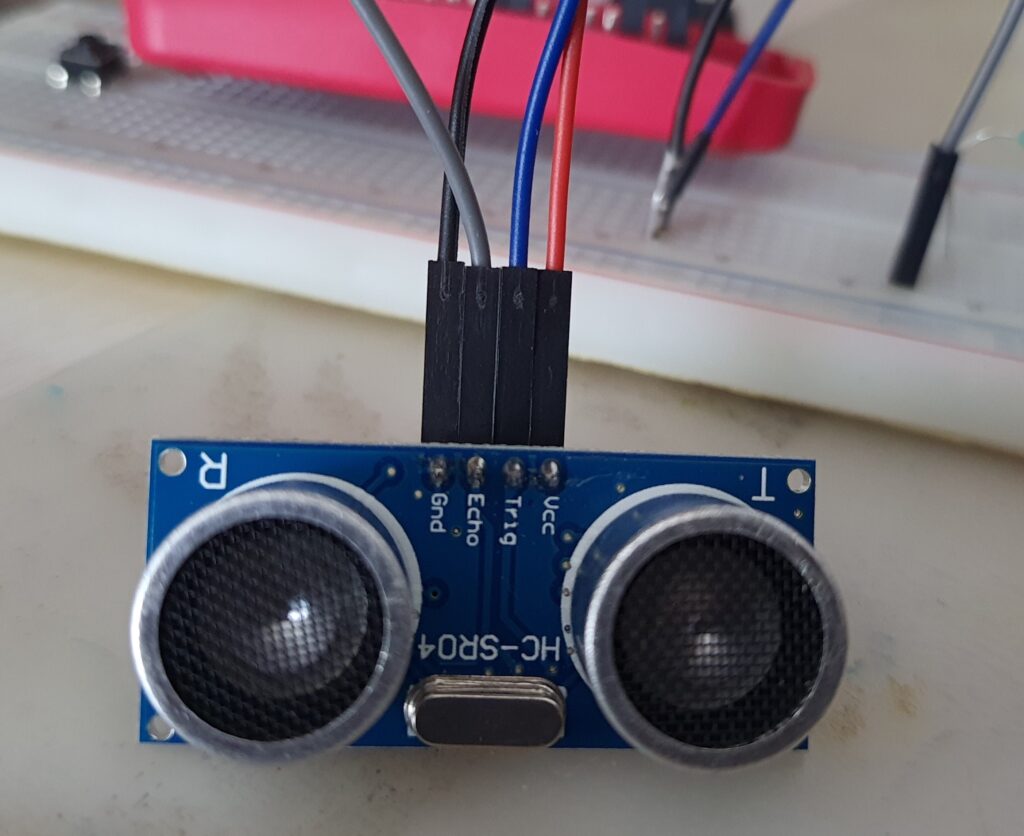
Working
We are going to power the module from raspberry pi. We will use our Raspberry Pi to send an input signal to TRIG, this will triggers the sensor to send an ultrasonic rays. The UV waves will bounce off any nearby objects and some are reflected back to the sensor. The sensor will detects these return waves and measures the time between the trigger and returned waves, and then sends a 5V signal on the ECHO pin.
ECHO will be “low” (0V) until the sensor is triggered when it receives the echo waves. Once a return wave is received ECHO is set as “high” (5V) for the duration of that wave. Our Python script will measure the wave duration and then calculate distance from this.
IMPORTANT. The sensor output signal (ECHO) on the HC-SR04 is rated at 5V. But, the input pin on the Raspberry Pi GPIO is rated at 3.3V. Sending a 5V signal into that unprotected 3.3V input port could damage your GPIO pins. Hence to avoid this we need to use a small voltage divider circuit, consisting of two resistors, to lower the sensor output voltage to something our Raspberry Pi can handle. To get the value of Resistance of voltage divider we can use the formula
Vout=Vin * ( R2/R1+R2)
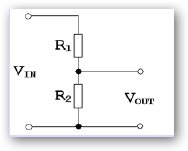
Refer my old post on basic electronics for details.
What you need?
- HC-SR04
- 1.5kΩ Resistor
- 2.2 kΩ Resistor
- Jumper Wires
- Any Raspberry pi( I am using Pi Zero W)
Assemble the circuit as follows
I will be using GPIO pin 23 and 24 on the Raspberry Pi for this project:
- GPIO 5V [Pin 2]————- Vcc (5V Power)
- GPIO GND [Pin 6] ——— GND (0V Ground),
- GPIO 23 [Pin 16] ———- TRIG (GPIO Output) and
- GPIO 24 [Pin 18] ———- ECHO (GPIO Input) via voltage divider
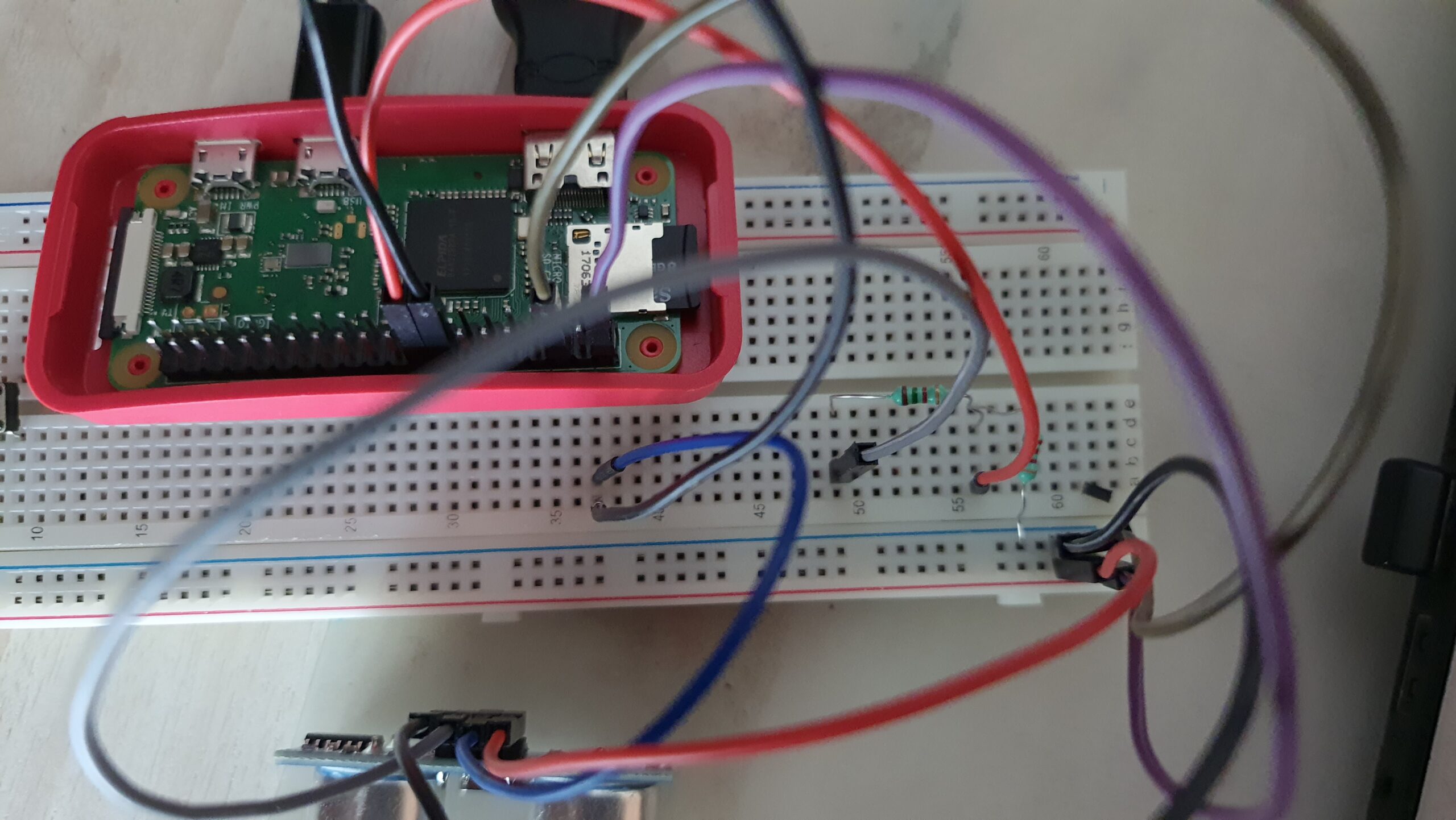
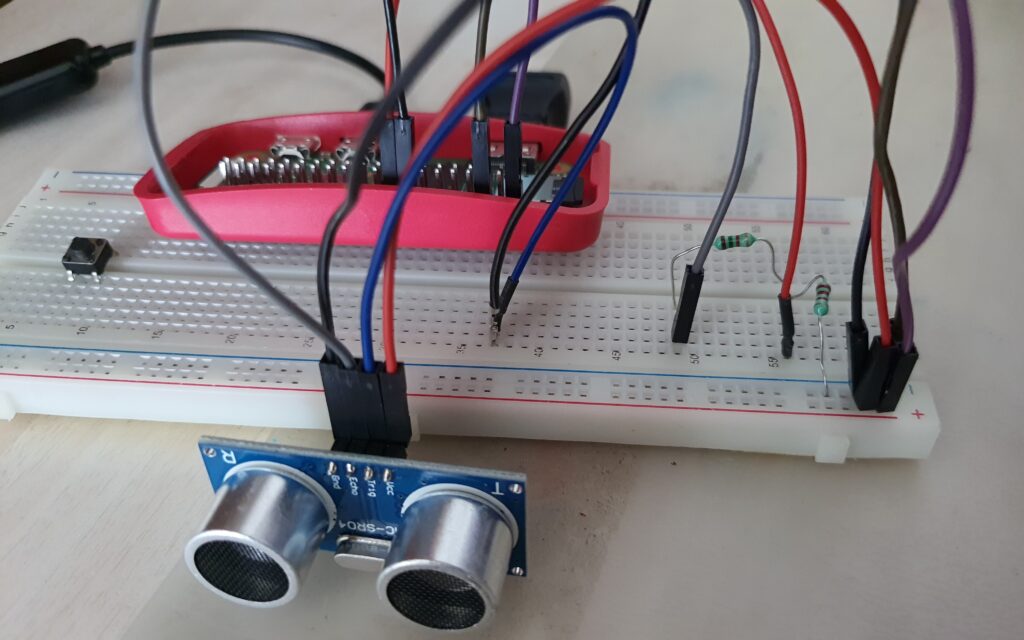
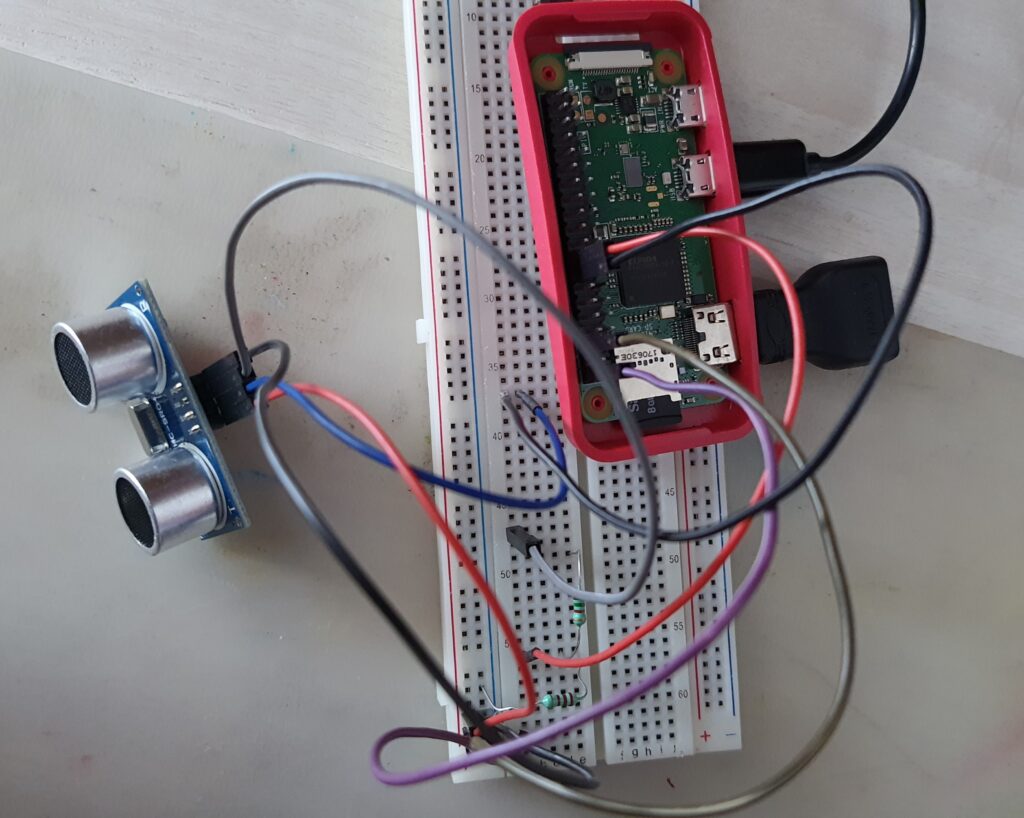
Python Code
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
#set GPIO Pins
GPIO_TRIGGER = 23
GPIO_ECHO = 24
#set GPIO direction (IN / OUT)
GPIO.setup(GPIO_TRIGGER, GPIO.OUT)
GPIO.setup(GPIO_ECHO, GPIO.IN)
def distance():
# set Trigger to HIGH
GPIO.output(GPIO_TRIGGER, True)
# set Trigger after 0.01ms to LOW
time.sleep(0.00001)
GPIO.output(GPIO_TRIGGER, False)
StartTime = time.time()
StopTime = time.time()
# save StartTime
while GPIO.input(GPIO_ECHO) == 0:
StartTime = time.time()
# save time of arrival
while GPIO.input(GPIO_ECHO) == 1:
StopTime = time.time()
# time difference between start and arrival
TimeElapsed = StopTime - StartTime
# multiply with the sonic speed (34300 cm/s)
# and divide by 2, because there and back
distance = TimeElapsed * 17150
distance = round(distance=1.15,2)
return distance
if __name__ == '__main__':
try:
while True:
dist = distance()
print ("Measured Distance = %.1f cm" % dist)
time.sleep(2)
# Reset by pressing CTRL + C
except KeyboardInterrupt:
print("Measurement stopped by User")
GPIO.cleanup()