In the previous post we have seen how to write unit test using unittest framework in Python. Here we will extend it and use for Selenium test automation using Python.
Prerequisite
Install Selenium driver binding for Python using following command
pip install Selenium
Sample Code
import unittest
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
class SeleniumBase(unittest.TestCase):
#url='https://www.amazon.in/'
def setUp(self):
self.driver=webdriver.Chrome()
def test_search_amazon(self):
driver=self.driver
driver.get("https://www.amazon.in/")
searchid=driver.find_element(By.ID,'twotabsearchtextbox')
searchid.send_keys('headphones')
searchid.send_keys(Keys.RETURN)
self.assertNotIn("No Results Found",driver.page_source)
def test_search_amazon_noresult(self):
driver=self.driver
driver.get("https://www.amazon.in/")
searchid=driver.find_element(By.ID,'twotabsearchtextbox')
searchid.send_keys('jdsfkjajka')
searchid.send_keys(Keys.RETURN)
result=driver.find_element(By.CLASS_NAME,'a-row')
self.assertEquals("No results for jdsfkjajka.",result.text)
def tearDown(self):
self.driver.close()
self.driver.quit()
if name == 'main':
unittest.main()
In the above example we have used setUp and tearDown of unittest method. The code is self explanatory if you have used selenium previously.
There is 2 test method starting with test keyword. Below is the output of the test method.
Key things to note:
- import of unittest
- import of Selenium webdriver, By and Keys class
- setUp – initializing driver class
- tearDown – Closing and quitting the browser.
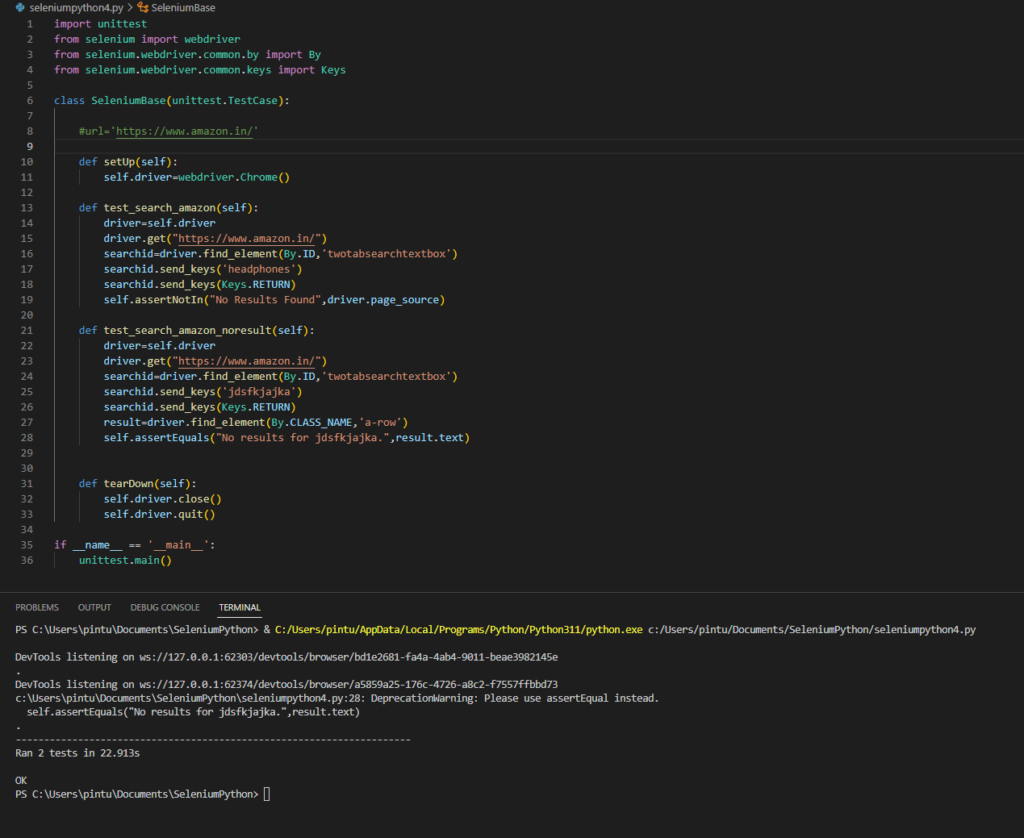
For more details on selenium go to official documentation here.