In this post we will see test automation using python and pytest. Previously we have seen how to write Selenium test using Python and unittest. Python unittest has many advantages like:
- Simple and easy to use
- Comprehensive reporting- Python unittest framework provides comprehensive reporting features, which allows developers to easily analyze and debug the test results.
- Integration with Selenium – Python unittest framework can be easily integrated with Selenium, which allows developers to test web applications with ease. This integration enables the use of Selenium WebDriver API and provides access to various browser options and capabilities.
- Automated testing – Python unittest framework supports automated testing, which saves time and effort by executing the test cases automatically. This feature is especially useful for regression testing, where a large number of test cases need to be executed repeatedly.
Though unittest has many advantages, there are many drawbacks of using it for Selenium test autoation
- Limited features: Python unittest framework has limited features compared to other testing frameworks. It lacks some advanced features like parallel test execution and distributed testing, which can be important for testing large-scale applications.
- Maintenance overhead: Maintaining test cases can be time-consuming and challenging, especially when the application being tested undergoes frequent changes.
Some alternative to unittest are pytest, Robot framework, Behave etc.
Using pytest
Install pytest if not already done using following command
pip install pytest
Let’s say we have a simple Python function that adds two numbers as shown below:
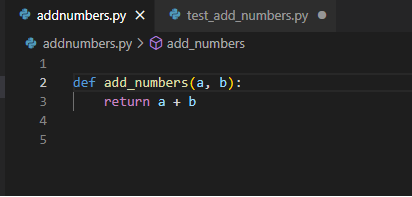
We can write a test case for this function using Pytest.
- Create a new Python file called test_add_numbers.py
- Import the add_numbers function from the module where it is defined
- Write a test function that tests the add_numbers function. The function should start with the prefix test_
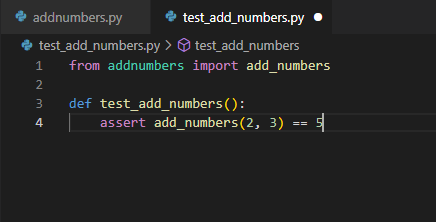
Run the Pytest command in the terminal to execute the test
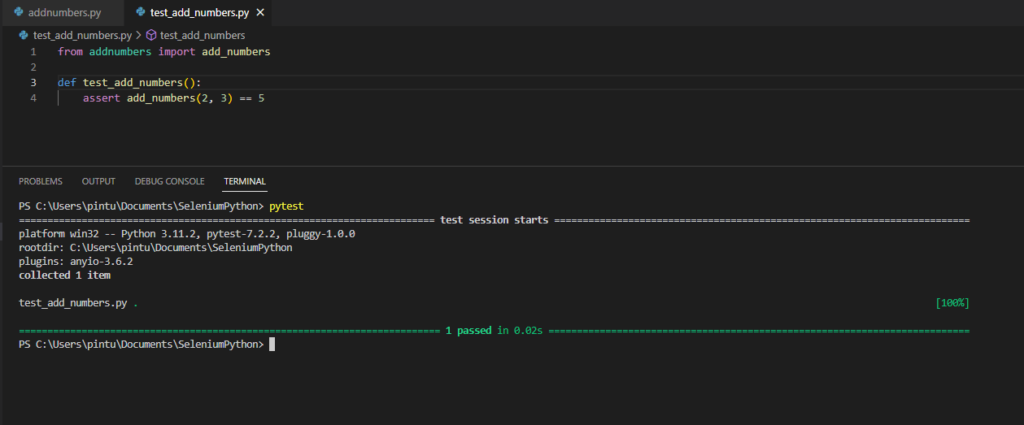
Selenium example
import pytest
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
def test_amazon_search():
# Initialize the webdriver
driver = webdriver.Chrome()
# Navigate to Amazon.in
driver.get("https://www.amazon.in/")
# Find the search box and enter a search term to search for any item
searchid=driver.find_element(By.ID,'twotabsearchtextbox')
searchid.send_keys('headphones')
# Press Enter to submit the search
searchid.send_keys(Keys.RETURN)
# Wait for the search results to load
driver.implicitly_wait(10)
# Assert that the search results page title contains the search term
assert "headphones" in driver.title
# Quit the webdriver
driver.quit()
Run the test as Pytest. It will show result as below.
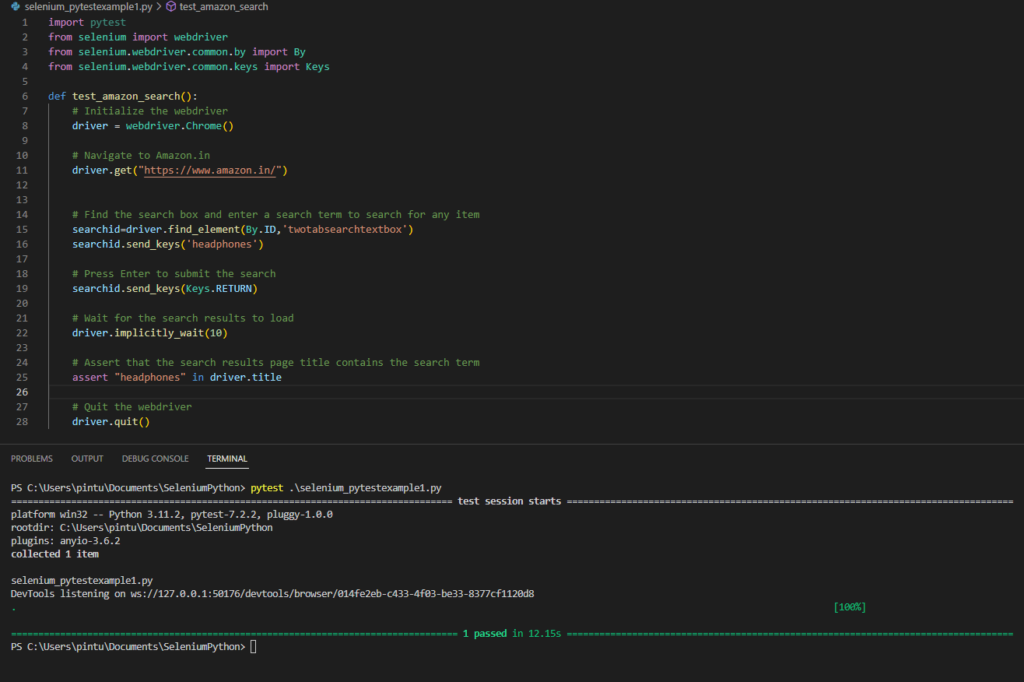
Parallel Selenium tests in Python
To write and run parallel Selenium tests in Python, you can use a combination of Selenium Grid, Pytest, and pytest-xdist plugin.
- Set up Selenium Grid: You will need to set up a Selenium Grid that allows you to run tests on multiple browsers and platforms in parallel. You can use Docker to set up the Selenium Grid
- Install pytest-xdist plugin: You can install them using pip:
pip install pytest pytest-xdist
- Write your test cases: Write your Selenium test cases using the WebDriver API. You can organize your test cases using Pytest fixtures and functions.
- Use pytest-xdist to run tests in parallel: Use the pytest-xdist plugin to run your test cases in parallel. The pytest-xdist plugin allows you to specify the number of CPUs or worker nodes to use for test execution.
pytest -n <number_of_workers>
eg: pytest -n 2
This will distribute your test cases across two worker nodes and run them in parallel.
You can also use other options provided by the pytest-xdist plugin, such as –boxed to run each test in a separate process, or –looponfail to continuously monitor your tests and rerun failed tests.